Chapter 3 of Effective TypeScript covers type inference: the process by which TypeScript infers the type of symbols in the absence of explicit annotations. A significant fraction of the comments I leave on TypeScript code reviews point out places where type annotations are unnecessary and can be omitted. This item explains why explicitly annotating inferable types is typically a bad idea, and enumerates a few specific exceptions to this rule.
The first thing that many new TypeScript developers do when they convert a codebase from JavaScript is fill it with type annotations. TypeScript is about types, after all! But in TypeScript many annotations are unnecessary. Declaring types for all your variables is counterproductive and is considered poor style.
Don’t write:
|
Instead, just write:
|
If you mouse over x
in your editor, you’ll see that its type has been inferred as number
:
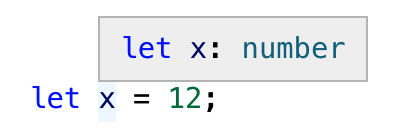
The explicit type annotation is redundant. Writing it just adds noise. If you're unsure of the type, you can check it in your editor.
TypeScript will also infer the types of more complex objects. Instead of:
|
you can just write:
|
Again, the types are exactly the same. Writing the type in addition to the value just adds noise here. (Item 21, Understand Type Widening, has more to say on the types inferred for object literals.)
What's true for objects is also true for arrays. TypeScript has no trouble figuring out the return type of this function based on its inputs and operations:
|
TypeScript may infer something more precise than what you expected. This is generally a good thing. For example:
|
"y"
is a more precise type for the axis
variable, and using it may fix some errors that would appear with the less-precise string
.
Allowing types to be inferred can also facilitate refactoring. Say you have a Product
type and a function to log it:
|
At some point you learn that product IDs might have letters in them in addition to numbers. So you change the type of id
in Product
. Because you included explicit annotations on all the variables in logProduct
, this produces an error:
|
Had you left off all the annotations in the logProduct
function body, the code would have passed the type checker without modification.
A better implementation of logProduct
would use destructuring assignment:
|
This version allows the types of all the local variables to be inferred. The corresponding version with explicit type annotations is repetitive and cluttered:
|
Explicit type annotations are still required in some situations where TypeScript doesn’t have enough context to determine a type on its own. You have seen one of these before: function parameters.
Some languages will infer types for parameters based on their eventual usage, but TypeScript does not. In TypeScript, a variable's type is generally determined when it is first introduced.
Ideal TypeScript code includes type annotations for function/method signatures but not for the local variables created in their bodies. This keeps noise to a minimum and lets readers focus on the implementation logic.
There are some situations where you can leave the type annotations off of function parameters, too. When there’s a default value, for example:
|
Here the type of base
is inferred as number
because of the default value of 10
.
Parameter types can usually be inferred when the function is used as a callback for a library with type declarations. The declarations on request
and response
in this example using the express HTTP server library are not required:
|
For much more on this, see Item 26: Understand How Context Is Used in Type Inference.
There are a few situations where you may still want to specify a type even where it can be inferred.
One is when you define an object literal:
|
When you specify a type on a definition like this, you enable excess property checking. This can help catch errors, particularly for types with optional fields. (This is discussed in more detail in Item 11: Recognize the Limits of Excess Property Checking.)
You also increase the odds that an error will be reported in the right place. If you leave off the annotation, a mistake in the object's definition will result in a type error where it's used, rather than where it's defined:
|
With an annotation, you get a more concise error in the place where the mistake was made:
|
Similar considerations apply to a function's return type. You may still want to annotate this even when it can be inferred to ensure that implementation errors don't leak out into uses of the function.
Say you have a function which retrieves a stock quote:
|
You decide to add a cache to avoid duplicating network requests:
|
There’s a mistake in this implementation: you should really be returning Promise.resolve(cache[ticker])
so that getQuote
always returns a Promise. The mistake will most likely produce an error… but in the code that calls getQuote
, rather than in getQuote
itself:
|
When you annotate the return type, it keeps implementation errors from manifesting as errors in user code. (async
functions are an effective way to avoid this specific error with Promises. They're discused in detail in Item 25: Use async Functions Instead of Callbacks for Asynchronous Code).
Writing out the return type may also help you think more clearly about your function: you should know what its input and output types are before you implement it. While the implementation may shift around a bit, the function's contract (its type signature) generally should not. This is similar in spirit to test-driven development (TDD), in which you write the tests that exercise a function before you implement it. Writing the full type signature first helps get you the function you want, rather than the one the implementation makes expedient.
A final reason to annotate return values is if you want to use a named type. You might choose not to write a return type for this function, for example:
|
TypeScript infers the return type as { x: number; y: number; }
. This is compatible with Vector2D
, but it may be surprising to users of your code when they see Vector2D
as a type of the input and not of the output:

If you annotate the return type, the presentation is more straightforward. And if you've written documentation on the type (Item 48: Use TSDoc for API Comments) then it will be associated with the returned value as well. As the complexity of the inferred return type increases, it becomes increasingly helpful to provide a name.
If you are using a linter, the eslint rule no-inferrable-types
(note the variant spelling) can help ensure that all your type annotations are really necessary.
Things to Remember
- Avoid writing type annotations when TypeScript can infer the same type.
- Ideally your code has type annotations in function/method signatures but not on local variables in their bodies.
- Consider using explicit annotations for object literals and function return types even when they can be inferred. This will help prevent implementation errors from surfacing in user code.